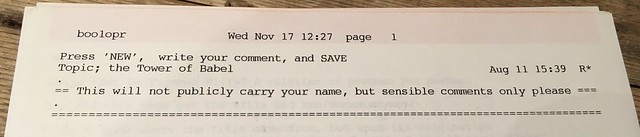
Back in the 1990s, the BBC World Service had a newsroom computer system called EDiT. This was the World Service version of a system called BASYS which had a bit of an interesting past. It was widely used in TV and radio newsrooms all over the world, used by domestic BBC TV and radio as well as CNN and ITN and I think maybe the ABC in Australia as well. Indeed, ITN actually owned BASYS / Newsfury. [See ITN staff magazine The Lens, issue 7, January 1994, page 7, 'ITN steps in to buy share of 'Newsfury' potential] As I recall this was because they’d invested heavily in BASYS equipment and the company got into financial difficulty and they bought it out to protect their investment. This new technology was controversial at the time, as this article from 2013 by Rory Cellan-Jones attests, but journalists and PAs would regard it with nostalgia when it was later replaced by ENPS, the Associated Press’s ‘Electronic News Production System’ or ‘Every News Programme Suffers’ as it was briefly known.
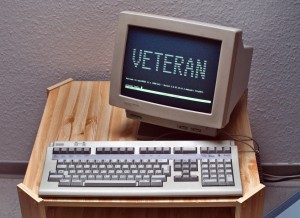
DEC VT420 terminal, by Jacek Rużyczka - Self-photographed, CC BY-SA 3.0, https://commons.wikimedia.org/w/index.php?curid=6762692
It’s very hard to find anything about BASYS on the internet, not helped by the fact that the name is now used by an FPGA board and most BASYS systems were probably taken out of service before the world wide web became popular. I’ve asked on Twitter if any old BBC or ITN hacks still have any training manuals for it, but to no avail. Part of me would love to rescue the system software and get it running on an emulator.
As I recall it ran on a mainframe, or maybe a VAX mini-computer, and the users had dumb serial terminals on their newsroom desks. In Bush House we had lovely DEC terminals, possibly VT-420s with paper white CRT monitors. I recall a sneaky trick was to type SCREEN and press enter and run away. This scrolled the current script in huge letters in an autocue style. Another hack was to gain access to the training system with CONNECT TRAIN, though I think that was disabled once it was realised this was getting abused.
To encourage people to get to know this new-fangled technology, the enlightened engineers set up an area called bush.graffiti – a kind of bulletin board where users could type anything pretty much anonymously. This was well before social media had been invented or anyone had any kind of internet access on BBC computers, so it proved very popular. Indeed I met my wife in bush.graffiti before an announcer by the name of Neil Sleat introduced us in real life by the fish tank in the Bush House Club. The file would show the user name and time of the last edit, which may or may not be the last person to comment in a document. My user name was boo1opr – first 3 letters of my surname, a number, then department code, opr for ‘operational resources’, the studio managers’ department. How we laughed at our Portuguese colleague whose username was pis1por. Many graffiti users adopted pseudonyms like Cottonmouth, United by Yucca and Yeboah’s Witness.
bush.graffiti had two long-running threads that stuck in my mind. One was ‘Strange Fridges’, a long Derek and Clive style riff on surreal objects and people that the authors claimed to have discovered at the backs of their fridges.

Another was The Tower of Babel. Bush House, broadcast in 42 languages, geddit? In this thread, people imagined that this building that was strange and labyrinthine enough in real life, was the basis of a text-based computer adventure game.
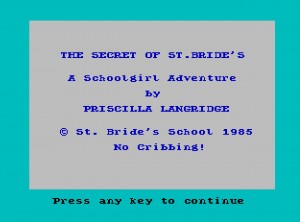
In lockdown, I got a bit obsessed with a couple of real adventure games. I’ve never got on with them in the past, always getting stuck and losing patience with, say, The Hobbit on the ZX Spectrum and the Hitch-Hiker’s Guide to the Galaxy PC game. Even now I gave up on the original Colossal Cave Adventure when I tried mapping it and realised that the geography was inconsistent and even changes during the game, which seems just maddeningly unfair. I went down a rabbit hole researching the, frankly quite astonishing, story of The Secret of St Bride’s and the enigmatic Games Mistresses who were behind it. They made well-regarded computer games but also ran a ‘school’ in Ireland which you could visit and stay at, where the staff all stayed in character, and yes that was probably every bit as weird and dodgy as it sounds. The main public face of the group may have been played by different people at different times, and any attempts to discover the truth about them seem to end in failure, madness or both. You can read more about the game and the people behind it here, but I warn you now, it won’t end well for you.
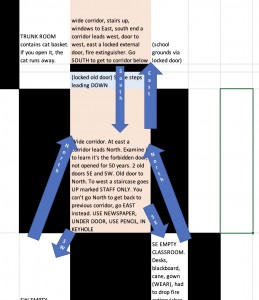
I tried mapping out the world of St Bride’s School in a spreadsheet, and even here was exasperated that going east from one space to another doesn’t necessarily mean you can return by going west. There’s also an infuriating point I would never have been able to get past back in the 80s without the internet’s help: assuming you know what to do and have gathered the right objects, you have to use precisely the right phrases USE NEWSPAPER, UNDER DOOR, USE PENCIL, IN KEYHOLE to progress. 1985 me would have given up in frustration at that point.
So, a mere thirty years after contributing to bush.graffiti, I decided to right those wrongs and make The Tower of Babel into a real text-based adventure, or ‘interactive fiction’ as I think they’re now called. You can play it online here, read a short intro here and the cribsheet may be useful.
Your character is a trainee SM (studio manager) doing a nightshift in the year 1991. You’re woken in the dorm (yes Bush House used to have a dormitory) at 4am and have until 5am to get to a studio to put a programme on air. I’ve taken some liberties with geography, but it’s broadly as I remember it. Move around with n, s, e, w, use ‘get’ or ‘take’ to pick things up, ‘i’ for listing your inventory and examining things may be useful. You can even examine yourself, if that’s your bag. You’ll need to find a studio key as you’ve left yours at home and some rooms have gatekeepers who may be prone to bribery.

The game isn’t finished yet, but you can regard getting into the studio before the PTT (pre-transmission test) alarm goes off a success. I’ll add some stuff about getting the show on air next so you can actually use some of the things I’ve packed into the studio’s control cubicle.
I was going to create it as an old-school, authentic ZX Spectrum game using the Quill software many 1980s games were written in, but instead I decided to write it using Inform which means you can also download the game to play in the IF platform of your choice as well as playing in your web browser. Learning Inform has been almost as frustrating as playing an adventure game in its own right, and I warn you if you’re tempted to follow suit: it will MESS WITH YOUR MIND. If you decide to drink the drink and eat the mushroom, don’t come running to me for sympathy. Actually you can. I’ll even provide the tea.
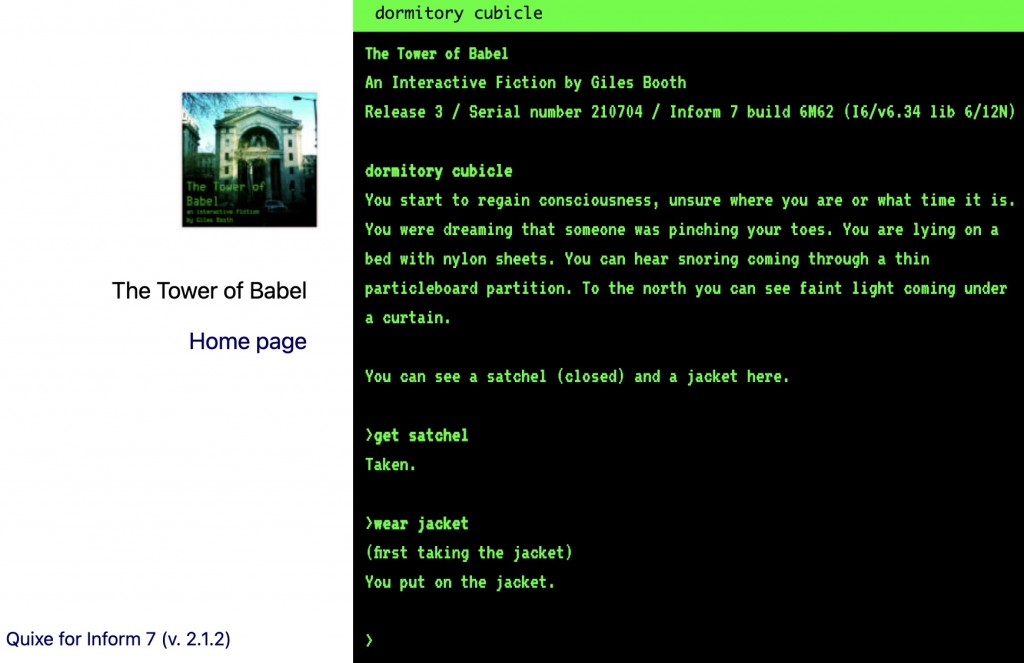
I think the game is playable as far as it goes if you know nothing about working in Bush House in the BBC World Service era, but if you were there you’ll find some in-jokes and you may be able to find some familiar parts of the building.
Whilst I appreciate this is incredibly niche to make an adventure game, sorry interactive fiction, about a job I did 30 years ago in a building the BBC doesn’t even occupy any more, it was a fun, if maddening, experience. I now have more respect for the authors of adventure games and understand some of the reasons for design decisions they made that seemed odd to me as a player. Though I do think my geography is consistent and I hope the game is playable without too much convoluted, unguessable syntax. Getting past the canteen is a pig, I admit, but I have left some clues.
If you’re an old BASYS sysadmin or trainer and have some documentation or, heaven forbid, some actual software, get in touch.
If you worked in Bush, or even of you didn’t, let me know if you like the game or where you get stuck. I may be bribable.
Postscript
In October 2022, BBC Northern Ireland produced a radio series called Assume Nothing which covered the real story behind St Bride’s. Warning: it’s much darker than I was expecting and not an easy listen, but essential if your interest has at any time been piqued by the Games Mistresses: https://www.bbc.co.uk/sounds/brand/m001dd9t
You can browse some, but by no means all, of my photos of Bush House by clicking on the photo below:
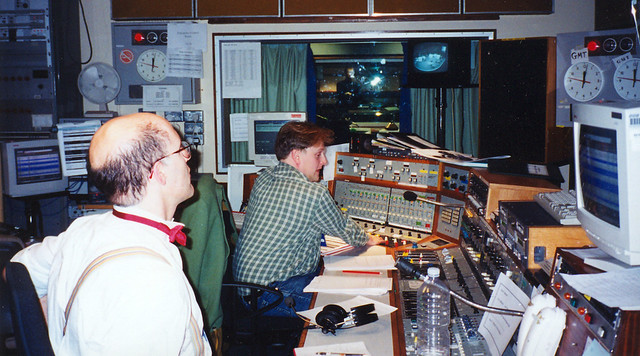